Building a Custom Analytics Dashboard for Your Dev Tool
Build a custom analytics dashboard to track key metrics and user behavior in your developer tool
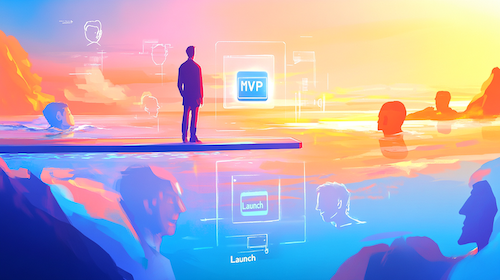
How PostHog Built Their First Analytics Dashboard
PostHog started with a simple problem: they needed to understand how developers used their open-source product analytics platform. Instead of using external tools, they built a basic dashboard using React and PostgreSQL that tracked just three metrics: daily active users, feature usage, and error rates. This focused approach helped them spot usage patterns that led to their first major product improvements.
Setting Up Your Analytics Foundation
Start with these core components:
1. Event tracking system
2. Data storage (PostgreSQL works well)
3. Data visualization layer
Essential Metrics to Track
Focus on these key indicators:
1. Daily/Weekly Active Users (DAU/WAU)
2. Feature adoption rates
3. Time-to-value measurements
4. Error rates and types
5. User paths and workflows
Building the Dashboard
Here's a basic React component structure:
import React from 'react';
import { LineChart, BarChart } from 'recharts';
const AnalyticsDashboard = () => {
const [activeUsers, setActiveUsers] = useState([]);
const [featureUsage, setFeatureUsage] = useState({});
useEffect(() => {
// Fetch your analytics data
fetchAnalyticsData().then(data => {
setActiveUsers(data.activeUsers);
setFeatureUsage(data.featureUsage);
});
}, []);
return (
);
};
Data Collection Strategy
Follow these principles for reliable data:
1. Track specific events, not page views
2. Use consistent event naming
3. Include relevant context with each event
4. Automate the collection process
Making Data Actionable
Transform raw data into insights:
1. Set up alerts for unusual patterns
2. Create weekly summary reports
3. Track retention indicators
4. Monitor feature adoption trends
Privacy and Security
Essential safeguards to implement:
1. Data anonymization
2. Secure storage practices
3. Clear data retention policies
4. User consent management
Remember that good analytics support better customer success. Your dashboard should help you make informed decisions about your product's direction.
Extra Tip: The One-Click Report
Create a script that generates a one-page PDF summary of your key metrics. This makes it easy to share insights with team members and stakeholders who don't need access to the full dashboard.
const generateReport = async () => {
const data = await fetchAnalyticsData();
const pdf = new PDFDocument();
pdf.text(`Weekly Report: ${new Date().toLocaleDateString()}`);
pdf.text(`Active Users: ${data.activeUsers.length}`);
pdf.text(`Top Features: ${Object.keys(data.featureUsage).join(", ")}`);
return pdf;
};
Start With Documentation
Create a simple system to document every support interaction. Use minimum viable processes to ensure consistency without overwhelming your team.
Build Support-Development Bridges
Set up regular meetings between support and development teams. Share support insights using customized dashboards to keep everyone aligned.
Test Solutions Quickly
Use feature flags to test solutions with small user groups before full rollout. This reduces risk and accelerates learning.
Measure Impact
Track how your solutions affect support volume and user satisfaction. Implement customer health scoring to measure improvement.
Start With Documentation
Create a simple system to document every support interaction. Use minimum viable processes to ensure consistency without overwhelming your team.
Build Support-Development Bridges
Set up regular meetings between support and development teams. Share support insights using customized dashboards to keep everyone aligned.
Test Solutions Quickly
Use feature flags to test solutions with small user groups before full rollout. This reduces risk and accelerates learning.
Measure Impact
Track how your solutions affect support volume and user satisfaction. Implement customer health scoring to measure improvement.
First Published:
Updated: