Using Python to Automate Your GitHub SEO: A Technical Guide
Learn how to automate GitHub repository optimization using Python for better visibility and discoverability
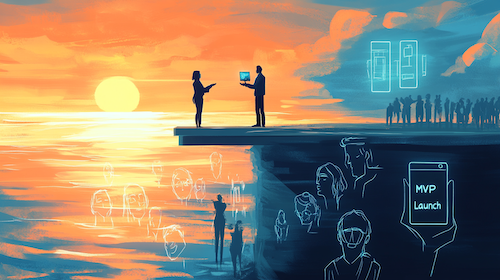
How Plausible Analytics Automated Their Way to 7k+ GitHub Stars
Plausible Analytics, an open-source alternative to Google Analytics, grew from 0 to 7,000+ GitHub stars by implementing automated repository optimization. Their founder Marko Saric wrote Python scripts to maintain consistent documentation, optimize README files, and update repository metadata regularly.
Setting Up Your Python Environment
First, you'll need these basics:
1. Python 3.7+
2. PyGithub library
3. GitHub personal access token
The Core Script
Here's a practical script to start with:
from github import Github
import os
def optimize_repo(repo_name, token):
g = Github(token)
repo = g.get_repo(repo_name)
# Update repository description
repo.edit(description="Your optimized description here")
# Update topics
repo.replace_topics(["python", "automation", "github", "seo"])
# Update README
update_readme(repo)
def update_readme(repo):
try:
contents = repo.get_contents("README.md")
readme_text = """
# Project Name
Clear description of what your project does
## Features
- Key feature 1
- Key feature 2
## Installation
```bash
pip install your-package
```
"""
repo.update_file(
contents.path,
"Update README with SEO optimizations",
readme_text,
contents.sha
)
except Exception as e:
print(f"Error updating README: {e}")
Key Areas to Automate
Focus your automation on these elements:
1. README file structure and content
2. Repository topics and tags
3. Repository description
4. Issue templates
5. Contributing guidelines
Implementing Automated Checks
Create checks for:
1. Missing documentation sections
2. Broken links
3. Image alt text
4. Code example completeness
By using smart automation, you're creating a foundation for better user retention. Good documentation and clear project structure help users stick around.
Monitoring and Analytics
Add these tracking elements:
1. Star count trends
2. Fork patterns
3. Clone statistics
4. Traffic sources
This data helps you understand user behavior and improve your repository accordingly.
Extra Tip: Engagement Hooks
Add a script to automatically tag and welcome first-time contributors. This personal touch increases engagement and encourages more contributions. Here's a sample code snippet:
def welcome_contributor(repo, pr):
if not has_previous_contributions(pr.user):
comment = f"Thanks @{pr.user.login} for your first contribution!"
pr.create_issue_comment(comment)
Start With Documentation
Create a simple system to document every support interaction. Use minimum viable processes to ensure consistency without overwhelming your team.
Build Support-Development Bridges
Set up regular meetings between support and development teams. Share support insights using customized dashboards to keep everyone aligned.
Test Solutions Quickly
Use feature flags to test solutions with small user groups before full rollout. This reduces risk and accelerates learning.
Measure Impact
Track how your solutions affect support volume and user satisfaction. Implement customer health scoring to measure improvement.
Start With Documentation
Create a simple system to document every support interaction. Use minimum viable processes to ensure consistency without overwhelming your team.
Build Support-Development Bridges
Set up regular meetings between support and development teams. Share support insights using customized dashboards to keep everyone aligned.
Test Solutions Quickly
Use feature flags to test solutions with small user groups before full rollout. This reduces risk and accelerates learning.
Measure Impact
Track how your solutions affect support volume and user satisfaction. Implement customer health scoring to measure improvement.
First Published:
Updated: