Building a Developer-Focused XML Sitemap Generator
Build an XML sitemap generator that developers will actually want to use - with code samples and best practices.
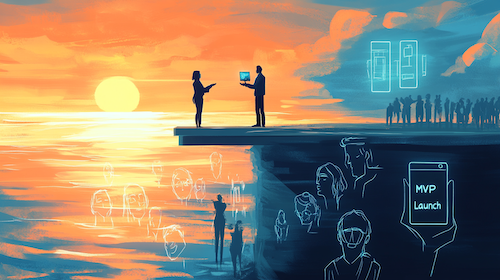
Why Build a Custom XML Sitemap Generator?
Most developers hit a wall when using standard sitemap generators - they don't handle dynamic routes, API documentation, or developer-specific content types well. This article shows you how to build a sitemap generator that puts developers first.
Real-World Impact
When Algolia needed to generate sitemaps for their documentation, they found standard tools lacking. Their solution? A custom generator that handled their specific needs, which later became an open-source tool helping countless other companies.
Key Requirements for Developer Sitemaps
A technical SEO testing framework needs specific features for developer content:
- Support for dynamic routes and API endpoints
- Handling of different documentation versions
- Integration with technical SEO for API docs
- Performance optimization for large documentation sets
Building the Generator
Let's create a generator that solves these challenges. We'll use Node.js for this example, but the principles apply to any language.
const fs = require('fs');
const path = require('path');
class DevSitemapGenerator {
constructor(config) {
this.baseUrl = config.baseUrl;
this.routes = [];
}
addRoute(route, options = {}) {
this.routes.push({
path: route,
priority: options.priority || 0.5,
changefreq: options.changefreq || 'weekly'
});
}
generateXml() {
// Implementation details
}
}
Handling Developer-Specific Cases
Your sitemap generator should handle these common scenarios:
- API reference pages with multiple versions
- Generated documentation from code comments
- Interactive examples and playgrounds
- Release notes and changelogs
Performance Considerations
When dealing with large documentation sets:
- Use streaming XML generation
- Implement efficient file system operations
- Cache intermediate results
- Break large sitemaps into smaller chunks
Integration Tips
Make your generator work seamlessly with:
- CI/CD pipelines
- Documentation build processes
- Content management systems
- Version control systems
Extra Tip: Handling Dynamic Routes
Create a route discovery system that automatically detects new documentation pages and API endpoints. This ensures your sitemap stays current without manual updates.
Recommended Next Steps
To make your sitemap generator more robust:
- Implement error logging and monitoring to track generation issues
- Add support for multiple output formats (XML, JSON, Text)
- Create a validation layer for URL structures
- Build in compression support for large sitemaps
Consider integrating with:
- Documentation testing tools
- Content management systems
- Deployment pipelines
- Custom analytics dashboards
Monitoring and Analytics
Track how search engines crawl your documentation:
- Monitor crawl rates and patterns
- Track indexed pages vs. submitted URLs
- Measure documentation page performance
Security Considerations
Protect sensitive documentation while maintaining SEO benefits:
- Exclude private API endpoints
- Handle authentication-required pages properly
- Manage rate limits and access controls
Future-Proofing Your Generator
Design your generator to handle growth:
- Support for new documentation types
- Extensible plugin system
- API versioning support
Common Myths About XML Sitemaps
Myth: Standard Sitemap Generators Are Good Enough
Reality: Developer documentation has unique needs that generic generators don't address.
Myth: Sitemaps Don't Impact API Documentation SEO
Reality: Well-structured sitemaps significantly improve the discovery and indexing of API documentation.
Myth: Building a Custom Generator Is Too Complex
Reality: Starting with a basic generator and iterating based on needs is a practical approach.
Sitemap Generator Readiness Check
Rate your current sitemap solution:
Taking Action
Ready to improve your documentation's SEO? Here's what to do next:
- Audit your current sitemap implementation
- Identify gaps in documentation coverage
- Start with a basic custom generator
- Implement monitoring and analytics
- Iterate based on crawl data
Share your progress and learn from others building similar tools in our community.
Join Our Developer Community
Building a sitemap generator? Don't do it alone:
- Join our X Community to connect with other developers tackling similar challenges
- List your sitemap generator on BetrTesters to get feedback and connect with potential users
- Share your implementation challenges and solutions with fellow developers
Your experience could help others improve their documentation SEO. Join us today and be part of the conversation!
Start With Documentation
Create a simple system to document every support interaction. Use minimum viable processes to ensure consistency without overwhelming your team.
Build Support-Development Bridges
Set up regular meetings between support and development teams. Share support insights using customized dashboards to keep everyone aligned.
Test Solutions Quickly
Use feature flags to test solutions with small user groups before full rollout. This reduces risk and accelerates learning.
Measure Impact
Track how your solutions affect support volume and user satisfaction. Implement customer health scoring to measure improvement.
Start With Documentation
Create a simple system to document every support interaction. Use minimum viable processes to ensure consistency without overwhelming your team.
Build Support-Development Bridges
Set up regular meetings between support and development teams. Share support insights using customized dashboards to keep everyone aligned.
Test Solutions Quickly
Use feature flags to test solutions with small user groups before full rollout. This reduces risk and accelerates learning.
Measure Impact
Track how your solutions affect support volume and user satisfaction. Implement customer health scoring to measure improvement.
First Published:
Updated: